My advent calendar didn’t have chocolate this year. Instead it had a Pico and lots of LEDs. Thanks to The Pi Hut for a very enjoyable project, and for the extensive guides which help a person get up and running with the Pico. All the code – micropython – in this post is provided by the Pi Hut, in their exceptionally usable and friendly daily guides that accompany the calendar.
My favourite two components were the 12-LED RGB Ring and the DHT20/AHT20 temperature and humidity sensor. And the actual temperature is shown on the 16×2 LCD (with I2C backpack). Apologies if the links are broken. I don’t expect the Pi Hut to keep them on sale forever, but I’m pretty sure you will be able to find something near enough.
While I enjoyed all the projects provided by the Pi Hut, the temperature sensor did strike a chord with me. I am a bit of a weather geek. I just love weather – especially snow. That’s why I made myself this temperature sensor. So I combined the projects from three different days, and made myself a thermometer which you can check from far – just to get an indication of the temperature, and you can check from close to get the actual temperature.
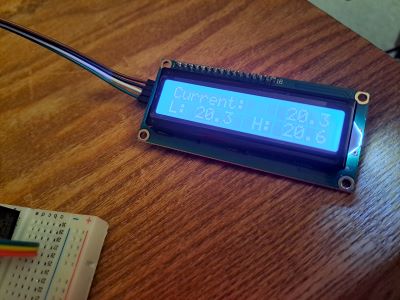
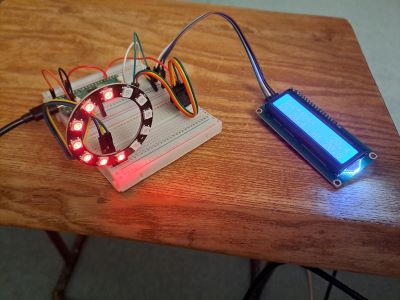
The idea is – when you are far away from the project, you can quickly see how many leds are lit. If more than half of the leds are lit, it is probably too warm, but if fewer are lit, it is probably too cool. In my case I decided 20 degrees was the ideal temperature, so when exactly half the leds are lit, the temperature is 20.
The code is all provided by the Pi Hut. I just combined code from a few different pages to get the final project that I wanted. And it is Micropython. I used Thonny which has a very nice interface with the Pico. And the wiring guides – you can probably get with each component you buy.
from machine import I2C, Pin
from lcd_api import LcdApi
from pico_i2c_lcd import I2cLcd
from dht20 import DHT20
from neopixel import NeoPixel
import time
# Define LCD/sensor I2C pins/BUS/address
SDA = 14
SCL = 15
I2C_BUS = 1
LCD_ADDR = 0x27
TEMP_ADDR = 0x38
# Define LCD rows/columns
LCD_NUM_ROWS = 2
LCD_NUM_COLS = 16
# Set up LCD I2C
lcdi2c = I2C(I2C_BUS, sda=machine.Pin(SDA), scl=machine.Pin(SCL), freq=400000)
lcd = I2cLcd(lcdi2c, LCD_ADDR, LCD_NUM_ROWS, LCD_NUM_COLS)
# Set up temperature sensor I2C
tempi2c = I2C(I2C_BUS, sda=SDA, scl=SCL)
dht20 = DHT20(TEMP_ADDR, tempi2c)
# Create a temperature/LED dictionary for our scale
# Temperature is the key (left), LED index is the value (right)
LEDdict = {
14: 0,
15: 1,
16: 2,
17: 3,
18: 4,
19: 5,
20: 6, # Top-middle LED (index 6 / LED #7) for 20°C
21: 7,
22: 8,
23: 9,
24: 10,
25: 11,
}
# Define the ring pin number (2) and number of LEDs (12)
ring = NeoPixel(Pin(2), 12)
# Write static LCD text
lcd.putstr("Current:")
lcd.move_to(0, 1) # Move to second row
lcd.putstr("L: H:")
#Take initial reading
measurements = dht20.measurements
# Create temp and humidity variables
# From initial readings
lowtemp = round(measurements['t'],1)
hightemp = round(measurements['t'],1)
# Write initial low temp value to LCD
lcd.move_to(3, 1)
lcd.putstr(str(lowtemp))
# Write initial high temp value to LCD
lcd.move_to(12, 1) # 12th column, 2nd row
lcd.putstr(str(hightemp))
while True:
# Grab data from the sensor dictionary
measurements = dht20.measurements
# Create variable for current temp
tempnow = round(measurements['t'],1)
# Update current temp on display
lcd.move_to(12, 0)
lcd.putstr(str(tempnow))
# If the lowest temp is HIGHER than current temp
if tempnow < lowtemp:
# Update the lowest recorded temp
lowtemp = tempnow
# Update the LCD data
lcd.move_to(3, 1) # 3rd column, 2nd row
lcd.putstr(str(lowtemp))
# If the highest temp is LOWER than current temp
if tempnow > hightemp:
# Update the highest recorded temp
hightemp = tempnow
# Update the LCD data
lcd.move_to(12, 1) # 12th column, 2nd row
lcd.putstr(str(hightemp))
# Create a rounded variable for the temperature
temperature = round(measurements['t'])
if temperature not in LEDdict:
pass
print("*** Out of temperature range ***")
else:
for key in LEDdict:
if key <= temperature:
ring[LEDdict[key]] = (10,0,0) # Light the index LED
else:
ring[LEDdict[key]] = (0,0,0)
ring.write() # Write the LED data
# Check every 5 seconds
time.sleep(5)